PJ on Development
Thoughts and babbling about software development. Visual Basic, .NET platform, web, etc.
Title: | Simple FTP Client |
Description: | A simple class to communicate with an FTP server. |
Author: | Paulo Santos |
eMail: | pjondevelopment@gmail.com |
Environment: | VB.NET 2005 (Visual Studio 2005) |
Keywords: | Network, FTP, FTP Client |
Simple FTP Client
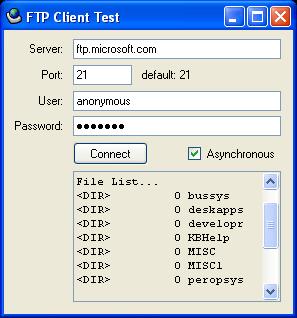
- Download Source Code - 43,90 KB
DISCLAIMERThe Software is provided "AS IS", without warranty of any kind, express or implied, including but not limited to the warranties of merchantability, fitness for a particular purpose and non-infringement. in no event shall the authors or copyright holders be liable for any claim, damages or other liability, whether in an action of contract, tort or otherwise, arising from, out of or in connection with the software or the use or other dealings in the software. |
||
Introduction
Experimenting with Visual Studio 2005 I discovered that .NET Framework 2.0 included a new class called FtpWebRequest
.
I was in need of a way to transfer some files programmatically to an FTP server, so I decided to take a closer look at this class.
The FtpWebRequest class is very straightforward to use, but it provides only the bare minimum to access an FTP server.
So I decided to roll up my sleeve and put something together in order to use this class and help me in my little project.
Creating the Class
Because I was just experimenting with this new class I decided to keep my implementation simple.
My class would only do four things on the FTP server:
- Retrieve the current directory.
- Upload a File
- Download a File
- Delete a File
Everything else at this time would be superfluous.
The only compromise I did against simplicity is that my class would perform all the operations both synchronous and asynchronously.
Synchronous and Asynchronous mode
Every method on the ftpClass is synchronous. The difference between both modes is that in the asynchronous mode events are fired in the beginning, during, and on the completion of an operation.
Future Improvements
There are thousands of items that I'd like to add in this class in the future. Among many others the main points are:
- Cancel an ongoing operation
- Ability to handle multiple Uploads and Downloads
- Resume broken Uploads and Downloads
- Better error handling and event definitions
- Multiple connections
- etc...
ftpClient Members
Public Enumerators
![]() | AttributeFlags A mask of bits that represents the permission for each file and folder on the FTP server. Public Enum AttributeFlags As UShort Directory = &H800 OwnerRead = &H100 OwnerWrite = &H80 OwnerExecute = &H40 GroupRead = &H20 GroupWrite = &H10 GroupExecute = &H8 PublicRead = &H4 PublicWrite = &H2 PublicExecute = &H1 None = 0 End Enum |
Public Constructors
![]() | ftpClient Constructor Initializes a new instance of the ftpClient Class. Public Sub New() Public Sub New(ByVal Server As System.Uri) Public Sub New(ByVal Server As System.Uri, _ ByVal UserCredentials As _ System.Net.NetworkCredential) Public Sub New(ByVal Server As String, _ ByVal Port As Integer) Public Sub New(ByVal Server As String, _ ByVal Port As Integer, _ ByVal UserName As String, _ ByVal Password As String) |
Public Properties
![]() | AllowUserInput Gets or sets a System.Boolean that specifies that in case of unknown FTP servers a window will be displayed to the user to request information in order to improve the FTP Library. Default True . Public Property AllowUserInput() As Boolean |
![]() | Async Gets of sets if the data transfer will be conducted asynchronously. Default False . Public Property Async() As Boolean |
![]() | BufferSize The size, in bytes, of the buffer used during data transfers. Default 1024 bytes. Public Property BufferSize() As Integer |
![]() | Busy ReadOnly. Indicates if the instance of the ftpClient class is transferring data. Public ReadOnly Property Busy() As Boolean |
![]() | FileList ReadOnly. An ftpDirectory instance that stores the list of files and folders of the current Path on the FTP server. Public ReadOnly Property FileList() As ftpDirectory |
![]() | Path The current path on the server. Public Property Path() As String |
![]() | Server A System.Uri instance that represents the FTP server. Public Property Server() As System.Uri |
![]() | StatusCode ReadOnly. A numeric code indicating the last response from the server. More information can be obtained in the RFC959. Public ReadOnly Property StatusCode() As _ System.Net.FtpStatusCode |
![]() | StatusDescription The human-readable description of the StatusCode. Public ReadOnly Property StatusDescription() _ As String |
![]() | UserCredentials A NetworkCredential instance that represents the FTP User Account used to log in the remote server. Public Property UserCredentials() As _ System.Net.NetworkCredential |
![]() | UseSSL Gets or sets a System.Boolean that specifies that an SSL connection should be used. Public Property UseSSL() As Boolean |
Public Methods
![]() | DeleteFile Deletes a file on the Server. After calling this method is necessary to call the Refresh method to ensure that the FileList property is up to date.Public Overloads Sub DeleteFile(ByVal FileName _ As String) Public Overloads Sub DeleteFile(ByVal File _ As ftpFile) |
![]() | DownloadFile Download a file from the FTP server. Public Overloads Sub DownloadFile( _ ByVal RemoteFileName As String, _ Optional ByVal LocalFileName As String = "") Public Overloads Sub DownloadFile( _ ByVal RemoteFileName As String, _ ByVal LocalStream As _ System.IO.Stream) Public Overloads Sub DownloadFile( _ ByVal RemoteFile As ftpFile, _ Optional ByVal LocalFileName As String = "") Public Overloads Sub DownloadFile( _ ByVal RemoteFile As ftpFile, _ ByVal LocalStream As _ System.IO.Stream)Note: When calling any of the overloaded DownloadFile method that accepts a Stream is the responsibility of the caller to close the stream upon the completion of the operation. |
![]() | Refresh Updates the FileList with fresh information from the FTP server. Public Sub Refresh() |
![]() | UploadFile Upload a file or a stream to the FTP server. Public Overloads Sub UploadFile( _ ByVal LocalFileName As String, _ Optional ByVal RemoteFileName As String = "") Public Overloads Sub UploadFile( _ ByVal LocalStream As _ System.IO.Stream, _ ByVal RemoteFileName As String)Note: When calling the overloaded UploadFile method that accepts a Stream is the responsibility of the caller to close the stream upon the completion of the operation. |
Public Events
![]() | onBeforeRequest Called before any request is made. Public Event onBeforeRequest( _ ByVal sender As Object, _ ByVal e As EventArgs) |
![]() | onRequestComplete Called on the completion of a request. Public Event onRequestComplete( _ ByVal sender As Object, _ ByVal e As EventArgs) |
![]() | onRequestProgress Called during request to inform the main thread of the progress. Public Event onRequestProgress( _ ByVal sender As Object, _ ByVal e As RequestProgressEventArgs) |
RequestProgressEventArgs
Public Properties
![]() | BytesReceived ReadOnly. The number of the bytes received on this request. Public ReadOnly Property BytesReceived() As Integer |
![]() | BytesSent ReadOnly. The number of the bytes sent on this request. Public ReadOnly Property BytesSent() As Integer |
![]() | BytesTotal ReadOnly. The total amount of bytes expected to be sent or received. Public ReadOnly Property BytesTotal() As Integer |
ftpDirectory Members
Inherits ArrayList
Public Properties
![]() | Item Returns an ftpFile object. Public Shadows Property Item(ByVal Index As Integer) _ As ftpFile |
ftpFile Members
Public Properties
![]() | Date The timestamp of the file. Public [Date] As DateTime |
![]() | Name The name of the file or folder. Public Name As String |
![]() | Owner The owner of the file or folder. If available. Public Owner As String |
![]() | Size The size of the file in bytes. Folders always have size 0. Public Size As ULong |
![]() | Flags A map of bits that represents the attributes of the file or folder. Public Property Flags() As AttributeFlags |
Public Methods
![]() | CanGroupExecute Returns a System.Boolean that indicates if the group can execute, read, or write the file. Public Function CanGroupExecute() As Boolean Public Function CanGroupRead() As Boolean Public Function CanGroupWrite() As Boolean |
![]() | CanOwnerExecute Returns a System.Boolean that indicates if the Owner can execute, read, or write the file. Public Function CanOwnerExecute() As Boolean Public Function CanOwnerRead() As Boolean Public Function CanOwnerWrite() As Boolean |
![]() | CanPublicExecute Returns a System.Boolean that indicates if the Public can execute, read, or write the file. Public Function CanPublicExecute() As Boolean Public Function CanPublicRead() As Boolean Public Function CanPublicWrite() As Boolean |
![]() | IsDirectory Returns a System.Boolean that indicates if this instance of ftpFile Class is a folder (or directory). Public Function IsDirectory() As Boolean |