PJ on Development
Thoughts and babbling about software development. Visual Basic, .NET platform, web, etc.
Title: | Ping Class |
Description: | How to ping a remote computer -- .NET Style |
Author: | Paulo Santos |
eMail: | pjondevelopment@gmail.com |
Environment: | VB.NET (1.0, 1.1, 2.0), Visual Studio (2002, 2003, 2005) |
Keywords: | Internet, Host, Ping |
Ping Class
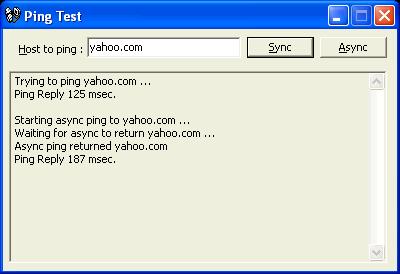
- PingClass v1.0 (2002) - 10,03 KB
- PingClass v1.1 (2003) - 10,92 KB
- PingClass v2.0 (2005) - 12,32 KB
DISCLAIMERThe Software is provided "AS IS", without warranty of any kind, express or implied, including but not limited to the warranties of merchantability, fitness for a particular purpose and non-infringement. in no event shall the authors or copyright holders be liable for any claim, damages or other liability, whether in an action of contract, tort or otherwise, arising from, out of or in connection with the software or the use or other dealings in the software. |
||
Introduction
This is an old class of mine. I created this class when I was developing a server monitoring system back in 2002, and I reasoned that the easiest way to know if the server was up is to ping it.
So, I started searching the web and I found a code developed for C# and after a few bumps I converted to VB .NET and the code works fine, up to the point of performance.
I use the Socket class to create a packet of raw data to be sent to the host. The send part works fine, but the response (when I listen for the echo of the packet I just sent) takes ages to process. I've checked the docs on Microsoft about the ReceiveFrom method to see if they have some insight about what's going on, and finally found a solution.
Since then I have published this code in some other places, always with good feedback from my fellow developers friends.
When I decided to publish it on my website I knew I needed to take another look at this class because so much time has passed.
One of the things that always bothered me with this class is that it was synchronous, i.e. when you call the method to ping the host it doesn't return until the host responds or a time-out is reached.
Hence I decided to go through it once more and make a second version of it with asynchronous methods too. This way is up to you to use the method that suits best your application.
Note:
In the .NET Framework 2.0 there is a namespace called NetworkInformation where a new class called Ping what supersedes this class.
PingClass Members
Public Enumerators
![]() | pingErrorCodes Enumerates the possible errors that might occur when pinging a remote host. Public Enum pingErrorCodes As Integer Success = 0 Unknown = (-1) HostNotFound = &H8001 SocketDidntSend = &H8002 HostNotResponding = &H8003 TimeOut = &H8004 BufferTooSmall = &H8101 DestinationUnreahable = &H8102 HostNotReachable = &H8103 ProtocolNotReachable = &H8104 PortNotReachable = &H8105 NoResourcesAvailable = &H8106 BadOption = &H8107 HardwareError = &H8108 PacketTooBig = &H8109 ReqestedTimedOut = &H810A BadRequest = &H810B BadRoute = &H810C TTLExprdInTransit = &H810D TTLExprdReassemb = &H810E ParameterProblem = &H810F SourceQuench = &H8110 OptionTooBig = &H8111 BadDestination = &H8112 AddressDeleted = &H8113 SpecMTUChange = &H8114 MTUChange = &H8115 Unload = &H8116 GeneralFailure = &H8117 End Enum |
Public Constructors
![]() | PingClass Constructor Initializes a new instance of the PingClass Class. Public Sub New() |
Public Properties
![]() | DataSize Gets or sets the size of the package to be send on the ping request. Default 32 bytes. Public Property DataSize() As Byte |
![]() | LastError ReadOnly. The last error occurred when pinging a host. Public ReadOnly Property LastError() _ As pingErrorCodes |
![]() | LocalHost ReadOnly. A System.Net.IPHostEntry that represents the local computer. Public ReadOnly Property LocalHost() _ As System.Net.IPHostEntry |
![]() | RemoteHost A System.Net.IPHostEntry that represents the remote host to be pinged. Public Property RemoteHost() _ As System.Net.IPHostEntry |
![]() | TimeOut The amount of time in milliseconds in which the remote host must reply. Public Property TimeOut() As Long |
Public Methods
![]() | BeginPing Begins the asynchronous execution of a PING request to a specified host. Public Overloads Shared Function BeginPing( _ ByVal fqdnHostName As String, _ ByVal requestCallback As PingCallback, _ ByVal stateObject As Object) _ As PingAsyncResult Public Overloads Shared Function BeginPing( _ ByVal ipAddress As System.Net.IPAddress, _ ByVal requestCallback As PingCallback, _ ByVal stateObject As Object) _ As PingAsyncResult |
![]() | EndPing Ends an asynchronous PING request. Public Shared Sub EndPing( _ ByVal par As PingAsyncResult) |
![]() | Ping Pings the remote host and return the number of milliseconds that the host took to reply. Public Overloads Function Ping() As Long Public Overloads _ Shared Function Ping( _ ByVal fqdnHostName As String) As Long Public Overloads _ Shared Function Ping( _ ByVal ipAddress As System.Net.IPAddress) As Long |
![]() | SetRemoteHost Sets the RemoteHost Property. Public Overloads Sub SetRemoteHost( _ ByVal fqdnHostName As String) Public Overloads Sub SetRemoteHost( _ ByVal ipAddress As System.Net.IPAddress) |
Using the code
For a quick and dirty sample just take a look at the follow snippet.
MsgBox("yahoo.com took " & _ PingClass.Ping("yahoo.com") & _ " milliseconds to reply")
Yes, it is that easy.
Background information
Always a good source of information about the Internet is the "Request For Comments". More specifically about ping and ping request are: